Version: Smart Feature Phone 2.5
Auth Assistant
Introduction
Auth Assistant extracts one-time passcode from sms and sends it to the caller app. It shows a loading page while expecting an message within a certain amount of time.
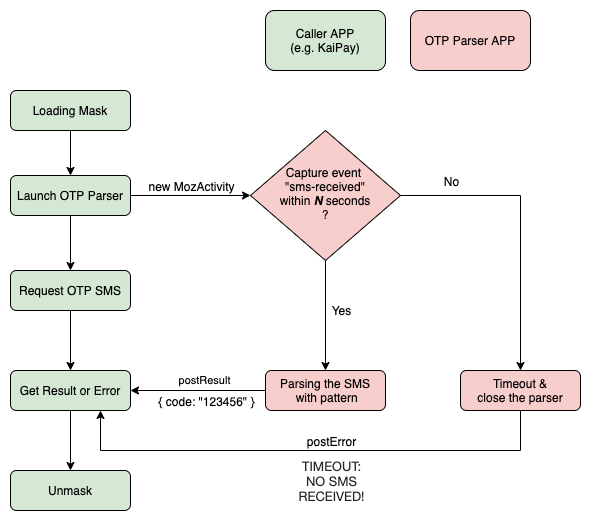
Activity restrictions
- pattern is required
- timeout must be greater than 10 seconds and less than 3 minutes (default to 30 seconds)
"filters": [
{
"type": "pattern",
"pattern": { "required": true }
}, {
"type": "timeout",
"timeout": { "required": false, "min": 10000, "max": 180000 }
}]
Testing Example
Example 1
Expected SMS message to match /KAIOS-([0-9]{4,8})/g
KAIOS-5566
Call
get-otp
activity with the corresponding message pattern
var activity = new MozActivity({
name: "get-otp",
data: {
pattern: /KAIOS-([0-9]{4,8})/g,
timeout: 60000
}
});
activity.onsuccess = function(evt) {
console.log("activity success. code: ", evt.target.result.code[0]);
};
activity.onerror = function() {
console.log(this.error);
};
- Send a message
navigator.mozMobileMessage.send(${phone_number}, "KAIOS-5566");
- Success message
activity success. code: KAIOS-5566
Example 2
Expected to match two patterns, /KAIOS-([0-9]{4,8})/g and /WW--([0-9]{4,8})/g
Your KaiOS account OTP code: KAI-5566 and WW-12345, please verify it within 2 minutes
Call
get-otp
activity with the corresponding message pattern
var activity = new MozActivity({
name: "get-otp",
data: {
pattern: [/KAIOS-([0-9]{4,8})/g, /WW-([0-9]{4,8})/g],
timeout: 120000
}
});
activity.onsuccess = function(evt) {
console.log(`activity success. code: ${evt.target.result.code[0]} and ${evt.target.result.code[1]}`);
};
activity.onerror = function() {
console.log(this.error);
};
- Send a message
navigator.mozMobileMessage.send(${phone_number}, "Your KaiOS account OTP code: KAI-5566 and WW-12345, please verify it within 2 min");
- Success message
activity success. code: KAI-5566 and WW-12345
Error handle
Device did not install Auth Assistant
- If device did not install Auth Assistant, the error callback function will be invoke.
NO_PROVIDER
var activity = new MozActivity({
name: 'get-otp',
data: {
pattern: /KAIOS-([0-9]{4,8})/g,
timeout: 10000,
},
});
activity.onsuccess = function (evt) {
console.log('activity success. code: ', evt.target.result.code[0]);
};
activity.onerror = function () {
console.log('activity error:', this.error.name);
if (this.error.name === 'NO_PROVIDER') {
// this activity is for directing user to install Auth Assistant
let storeActivity = new MozActivity({
name: 'inline-open-by-name',
data: {
type: 'name',
name: 'otpparser',
autoDownload: true,
},
});
}
};
- Error message
activity error: NO_PROVIDER
- You can use following code for redirect user to Store to install Auth Assistant.
var activity = new MozActivity({
name: 'get-otp',
data: {
pattern: /KAIOS-([0-9]{4,8})/g,
timeout: 10000,
},
});
activity.onsuccess = function (evt) {
console.log('activity success. code: ', evt.target.result.code[0]);
};
activity.onerror = function () {
console.log('activity error:', this.error.name);
if (this.error.name === 'NO_PROVIDER') {
// this activity is for directing user to install Auth Assistant
let storeActivity = new MozActivity({
name: 'inline-open-by-name',
data: {
type: 'name',
name: 'otpparser',
autoDownload: true,
},
});
}
};