Version: Smart Feature Phone 3.0
Auth Assistant
Introduction
Auth Assistant extracts one-time passcode from sms and sends it to the caller app. It shows a loading page while expecting an message within a certain amount of time.
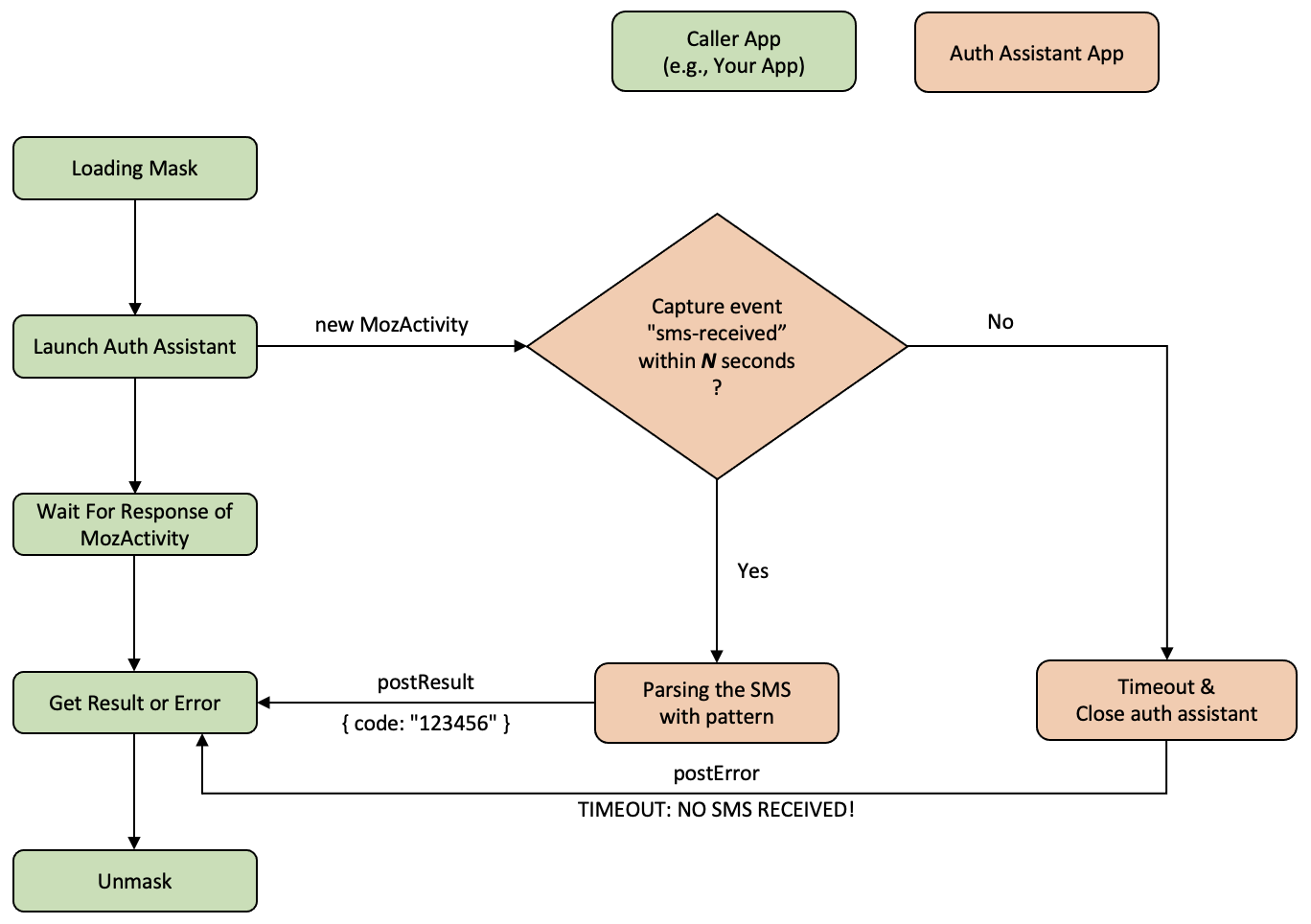
Activity restrictions
- pattern is required
- timeout must be greater than 10 seconds and less than 3 minutes (default to 30 seconds)
"filters": [
{
"type": "pattern",
"pattern": { "required": true }
}, {
"type": "timeout",
"timeout": { "required": false, "min": 10000, "max": 180000 }
}]
Testing Example
Example 1
- Expected SMS message to match /KAIOS-([0-9]{4,8})/g
KAIOS-5566
- Call
get-otp
activity with the corresponding message pattern
const activity = new WebActivity('get-otp', {
pattern: [/KAIOS-([0-9]{4,8})/g],
timeout: 10000,
});
activity.start().then(
r => {
console.log('activity success. code: ', r.code[0]);
},
e => {
console.log('activity error:', e)
}
);
- Send a SMS
navigator.b2g.mobileMessageManager.send(${phone_number}, 'KAIOS-5566')
- Success message
activity success. code: KAIOS-5566
Example 2
Expected to match two patterns, /KAIOS-([0-9]{4,8})/g and /WW--([0-9]{4,8})/g
Your KaiOS account OTP code: KAI-5566 and WW-12345, please verify it within 2 minutes
Call
get-otp
activity with the corresponding message pattern
const activity = new WebActivity('get-otp', {
pattern: [/KAIOS-([0-9]{4,8})/g, /WW-([0-9]{4,8})/g],
timeout: 120000,
});
activity.start().then(
r => {
console.log(`activity success. code: ${r.code[0]} and ${r.code[1]}`, );
},
e => {
console.log('activity error:', e)
}
);
- Send a SMS
navigator.b2g.mobileMessageManager.send(${phone_number}, "Your KaiOS account OTP code: KAI-5566 and WW-12345, please verify it within 2 min");
- Success message
activity success. code: KAI-5566 and WW-12345
Error handle
Time out
- If device did not receive SMS within timeout you set, the error callback function will be invoke.
TIMEOUT: NO SMS RECEIVED!
const activity = new WebActivity('get-otp', {
pattern: [/KAIOS-([0-9]{4,8})/g],
timeout: 60000,
});
activity.start().then(
r => {
console.log('activity success. code: ', r.code[0]);
},
e => {
console.log('activity error:', e)
}
);
- Error message
activity error: TIMEOUT: NO SMS RECEIVED!
Device did not install Auth Assistant
- If device did not install Auth Assistant, the error callback function will be invoke.
NO_PROVIDER
const activity = new WebActivity('get-otp', {
pattern: [/KAIOS-([0-9]{4,8})/g],
timeout: 60000,
});
activity.start().then(
r => {
console.log('activity success. code: ', r.code[0]);
},
e => {
console.log('activity error:', e)
}
);
- Error message
activity error: NO_PROVIDER
- You can use following code for redirect user to Store to install Auth Assistant.
const activity = new WebActivity('get-otp', {
pattern: [/KAIOS-([0-9]{4,8})/g],
timeout: 60000,
});
activity.start().then(
r => {
console.log('Activity Success', r.code[0]);
},
e => {
console.log('Activity Error', e);
if (e === 'NO_PROVIDER') {
// this activity is for directing user to install Auth Assistant
const storeActivity = new WebActivity('inline-open-by-name', {
type: 'name',
name: 'otpparser',
autoDownload: true,
});
storeActivity.start();
}
}
);